Get started integrating Rails 4 and QuickBooks Online with the Quickeebooks Gem: Part 3 : Disconnecting with QuickBooks
- Part 1: Make an OAuth connection between a Rails 4 app & QBO.
- Part 2: Create a Vendor on both Rails app and QBO.
- Part 3: Disconnecting from QBO.
- Part 4: Implementing Single Sign On.
- Code presented below is also available on Github
Table of Contents
Part 3: Disconnect the Rails app from QB0.
- Let's start by updating the view,
app/views/layouts/application.html.erb
, to show a disconnect link - Reload
- Make a new quickbooks_controller and move the
authenticate
andoauth_callback
actions from thevendors_controller
to here. - The new controller at
app/controllers/quickbooks_controller.rb
should look like the code below. Notice the highlighted sections that also need to be modified. - Modify
config/routes.rb
: - Then modify the following line in
app/views/layouts/application.html.erb
to reflect the new authenticate route. - Finally, make sure you issue a
touch tmp/restart.txt
to restart the app as the routes have changed. - Allow
set_qb_service
to create additional services. - Remove the
set_qb_service
fromvendors_controller
and move it toapplication_controller
and modify it as such: - Next, modify the
vendors_controller
instance vars of@vendor_service
to be just@service
- Reload
billtastic.dev/vendors
to test that the refactorings have taken effect with no errors. - Add the
disconnect
route. - Add the
disconnect
action to thequickbooks_controller
- Before we test disconnecting, let's log into the Intuit Partner
Platform like we did in Part
1. From there navigate to
My Apps
->Manage My Apps
and then to the Billtastic app. - Notice that you should have at least one live API connection
- While we are here let's also change the disconnect url to
billtastic.dev/quickbooks/disconnect
. - Let's go back to the Billtastic.app at
billtastic.dev/vendors
and click on the 'Disconnect from QuickBooks' link. - Yeah! A successfully disconnect.
- Let's make sure the disconnect occurred by checking over at the Intuit Partner Platform.
<% unless session[:token] %> <ipp:connectToIntuit></ipp:connectToIntuit> <% else %> <div> <%= link_to 'Disconnect from QuickBooks', '', data: { confirm: 'Are you sure you want to disconnect?'</div> <% end %>
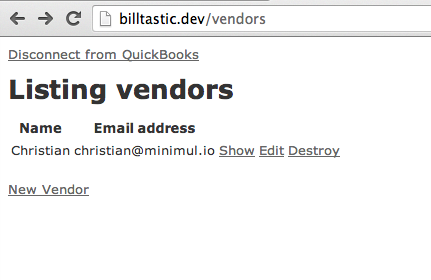
DISCUSSION: We need a
disconnect
action but the
vendors_controller
is getting crowded, therefore, let's
create the quickbooks_controller
. This is also a perfect place
for the authenticate
and oauth_callback
actions
so we are going to move them before creating the disconnect
action.
class QuickbooksController < ApplicationController def authenticate callback = oauth_callback_quickbooks_url token = $qb_oauth_consumer.get_request_token(:oauth_callback => callback) session[:qb_request_token] = token redirect_to("https://appcenter.intuit.com/Connect/Begin?oauth_token=#{token.token}") and return end def oauth_callback at = session[:qb_request_token].get_access_token(:oauth_verifier => params[:oauth_verifier]) session[:token] = at.token session[:secret] = at.secret session[:realm_id] = params['realmId'] flash.notice = "Your QuickBooks account has been successfully linked." @msg = 'Redirecting. Please wait.' @url = vendors_path render 'vendors/close_and_redirect', layout: false end end
Billtastic::Application.routes.draw do resources :vendors resources :quickbooks do collection do get :authenticate get :oauth_callback end end end
<script>
intuit.ipp.anywhere.setup({menuProxy: '/path/to/blue-dot', grantUrl: '<%= authenticate_quickbooks_url %>'});
</script>
DISCUSSION: We are still not done with the refactoring. The
disconnect
action
uses a different Quickeebooks service then from the one used in the
vendors controller so let's make the set_qb_service
method more
flexibility.
class ApplicationController < ActionController::Base
# Prevent CSRF attacks by raising an exception.
# For APIs, you may want to use :null_session instead.
protect_from_forgery with: :exception
private
def set_qb_service(type = 'Vendor')
oauth_client = OAuth::AccessToken.new($qb_oauth_consumer, session[:token], session[:secret])
@service = "Quickeebooks::Online::Service::#{type}".constantize.new
@service.access_token = oauth_client
@service.realm_id = session[:realm_id]
end
end
Billtastic::Application.routes.draw do
resources :vendors
resources :quickbooks do
collection do
get :authenticate
get :oauth_callback
get :disconnect
end
end
end
def disconnect set_qb_service('AccessToken') result = @service.disconnect if result.error_code == '270' msg = 'Disconnect failed as OAuth token is invalid. Try connecting again.' else msg = 'Successfully disconnected from QuickBooks' end session[:token] = nil session[:secret] = nil session[:realm_id] = nil redirect_to vendors_path, notice: msg end
DISCUSSION: The
disconnect
action
uses the AccessToken module/service from Quickeebooks. It provides a
disconnect
instance method that makes an API call requesting
the disconnect. Intuit states that an error_code of 0
indicates a successful request but I have found that an error_code of
'22'
worked fine and the connection was properly
disconnected. The session's are cleared in all cases because even on a
disconnect failure this indicates that the current credentials are invalid and
creating a new connection may be the solution.
IMPORTANT: There are many points were the Intuit v2 API doesn't work
exactly as stated in the docs as in the above example were I received an
error code of
'22'
but the disconnect worked fine. Don't beat
yourself up because you are not obtaining responses precisely as described in the
documentation. Just shrug your shoulders and move on.
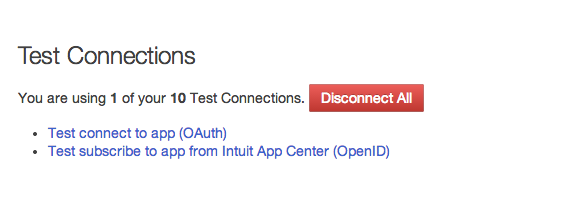


NOTE: This url change is not needed for this tutorial and is only
used for when a
disconnect
action is initiated from the Intuit
App Center. We want to make the change now while we are here and
the new disconnect route is fresh in our minds.
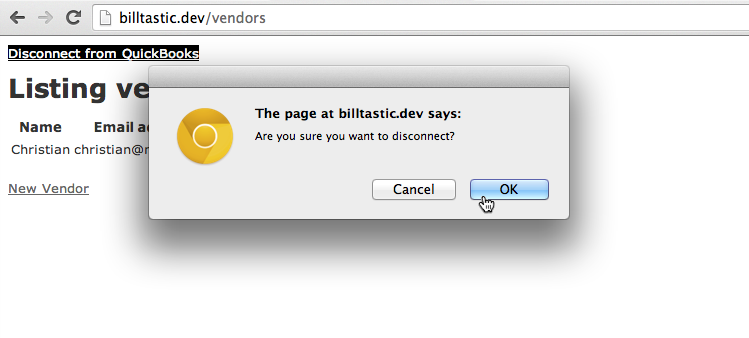
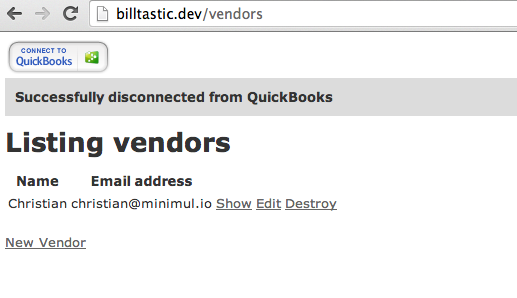
session[:token]
is nil.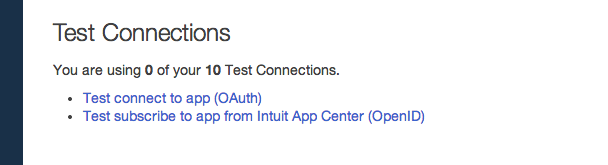
Implementing Single Sign On
Stay tuned to Part 4 in where I will hook up the Billtastic app with the ability to use your Intuit QBO credentials to sign on. This is referred to as "Single Sign On" by Intuit and is needed if you desire to have your application listed on their App Center.
- Pushed on 12/17/2013 by Christian
- QuickBooks Integration Consulting