Getting started with Nodejs and QuickBooks Online Part 4
Let's create a Sales Receipt!
In Part 4, I'll show how you can use the node-quickbooks's tests to create a SalesReceipt transaction against your developer sandbox. Specifically, I will be answering this question from the Intuit Developer forums.
- Make a new branch.
- Turn
on
debugging inconfig.js
. - Open up
test/index.js
. - Go down to the test starting with
describe('SalesReceipt', ..
. - Let's put this test that creates a sales receipt into its own test file
test/salesreceipt.js
. - Then let's apply the JSON request example in the question.
- Make a another test above the current one like this.
- Now let's run the test
- Let's use a
Service
type item so Item Id = 6, "Gardening" should work just fine. - Next, make sure you either delete the test from step 7 or mark it "skip".
- Run the test
$ git branch salesreceipt
- debug: false, + debug: true,
var os = require('os'),
fs = require('fs'),
util = require('util'),
expect = require('expect'),
async = require('async'),
_ = require('underscore'),
config = require('../config'),
QuickBooks = require('../index'),
qbo = new QuickBooks(config);
describe('SalesReceipt', function() {
this.timeout(30000);
it('should create a new SalesReceipt', function (done) {
var sr = {
"Line": [{
"Id": "1",
"LineNum": 1,
"Amount": 35.0,
"DetailType": "SalesItemLineDetail",
"SalesItemLineDetail": {
"ItemRef": {
"value": "61",
"name": "10 Pack Group X Classes"
},
"UnitPrice": 35,
"Qty": 1,
"TaxCodeRef": {
"value": "NON"
}
}
}]
}
qbo.createSalesReceipt(sr, function(err, salesReceipt) {
expect(err).toBe(null)
expect(salesReceipt.Fault).toBe(undefined)
done()
})
})
})
Ok, so we modified the test/salesreceipt.js
test with the JSON request from the forums question. But one glaring problem is the ItemRef: "61"
is not going to work because there is no Item with an Id of 61 in the sandbox. The Item Id 61 is coming from the users QBO.
Therefore, we need to find an adequate replacement from the developer sandbox but the sandbox UI doesn't show Ids for Items. The API does show ids so let's create a temporary "test" that will display sandbox items and their Ids.
// ... this.timeout(30000); it.only('find sandbox items', function (done) { qbo.findItems(function(_, items) { items.QueryResponse.Item.forEach(function(item) { console.log("id:" + item.Id + " name:" + item.Name + " type:" + item.Type) }) }) }); // ...
Notice, the it.only
syntax. These tests use the mocha
framework, which allows using only
so only this one test will run and all others will be skipped. Perfect for what we need here, which is simple request so we can plug in a valid ItemRef
Id to the other test.
$ npm test test/salesreceipt.js
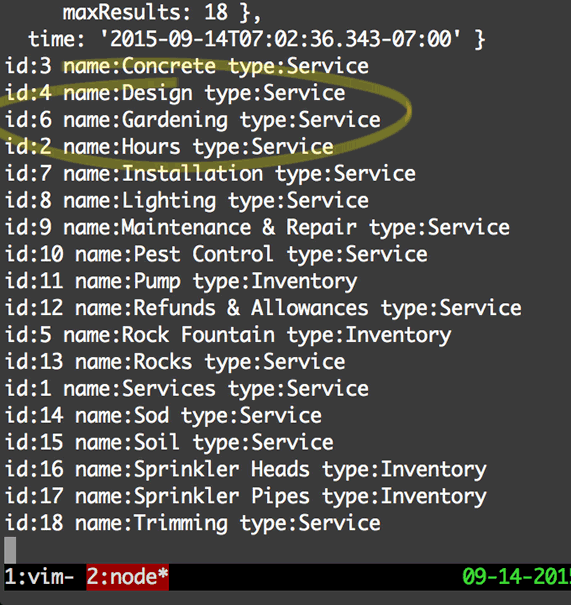
"ItemRef": { - "value": "61", + "value": "6", "name": "10 Pack Group X Classes" },
- it.only('find sandbox items', function (done) { + it.skip('find sandbox items', function (done) {
$ npm test test/salesreceipt.js
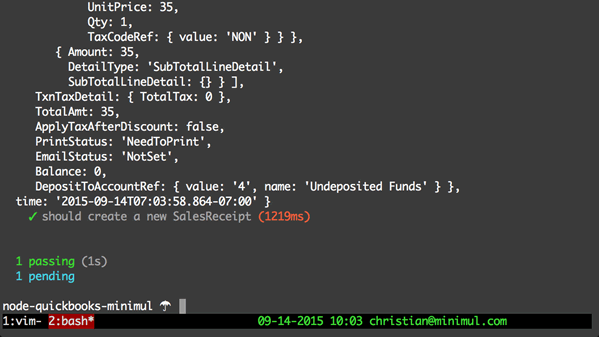
Conclusion
That's a wrap for this 4 part series, cumulating in creating a transaction entity in QuickBooks Online. Also, reference the code for this article as well as Part 3.
- Pushed on 09/17/2015 by Christian
- QuickBooks Integration Consulting