Connect to QuickBooks Angular directive
Simple Connect button rendering
I was attempting to answer this SO question which referenced this angular directive for rendering a "Connect to QuickBooks" button. I fired up the gulp-angular generator but couldn't get that directive to work and in the process was was able to come up with a simpler approach.
- Install the gulp-angular Yeoman generator, make a new dir, and run the generator.
- Install no additional items when prompted by the Yeoman wizard.
- Oops. The bower install failed.
- Re-run
bower install
. - Test the generator doing a
gulp serve
. - Let's clean up some of the scaffolding stuff. Remove the
awesomeThings
array insrc/app/main/main.controller.js
- Then remove the corresponding
ng-repeat
insrc/index.html
. - Make a new file
src/app/main/directives.js
and add the following. - Then in
src/index.html
do: - Let's check it out.
$ npm install -g generator-gulp-angular $ cd ~/github/labs $ mkdir connect-intuit-angular && cd $_ $ yo gulp-angular
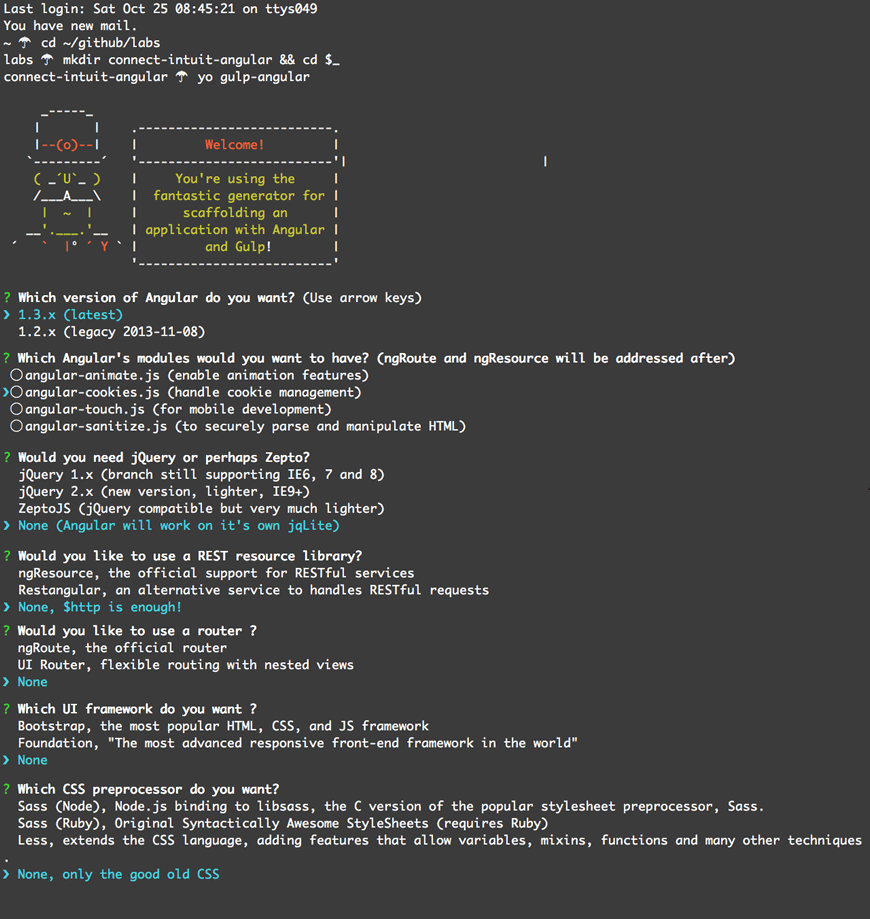
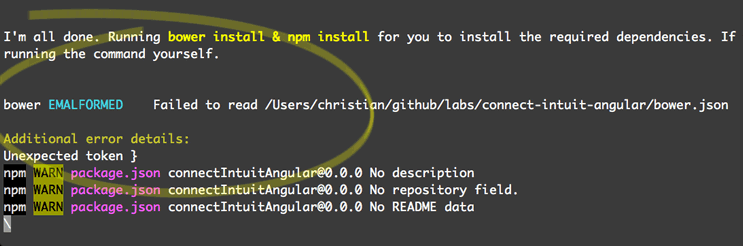
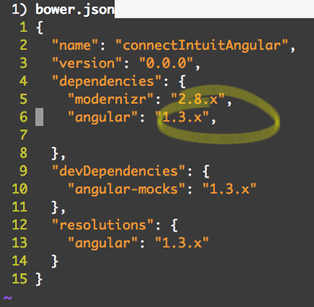
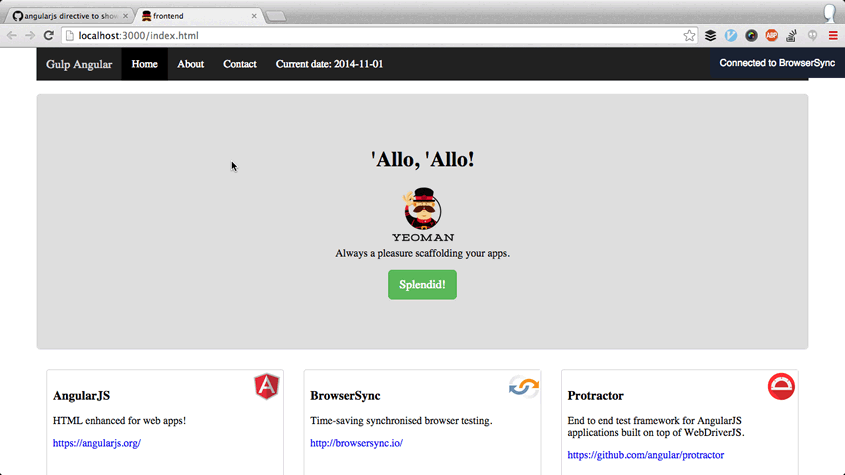
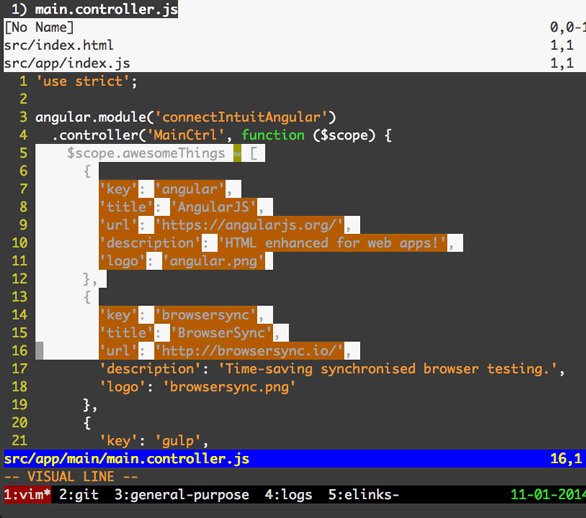
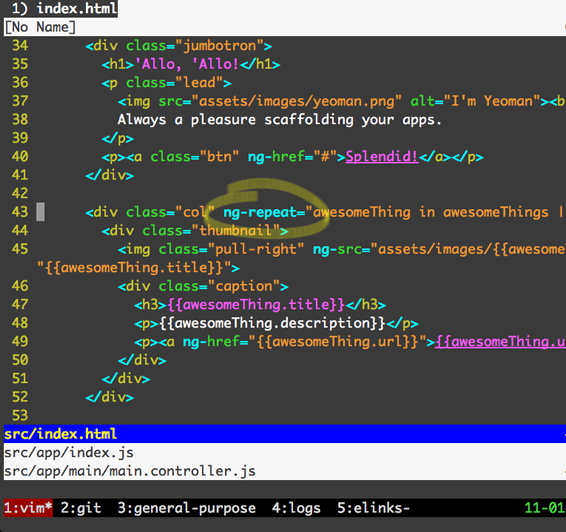
/* src/app/main/directives.js */ angular.module('connectIntuitAngular') .directive('connectToQuickbooks', function($window){ return { restrict: 'E', template: "<ipp:connectToIntuit></ipp:connectToIntuit>", link: function(scope) { var script = $window.document.createElement("script"); script.type = "text/javascript"; script.src = "//js.appcenter.intuit.com/Content/IA/intuit.ipp.anywhere.js"; script.onload = function () { scope.$emit('intuitjs:loaded'); }; $window.document.body.appendChild(script); scope.$on('intuitjs:loaded', function (evt) { $window.intuit.ipp.anywhere.setup({ grantUrl: '/' }); scope.connected = true; scope.$apply(); }); } } });
<!-- index.html --> <div class="jumbotron" ng-init="connected = false"> <div class="control-group"> <div class="controls" ng-show="!connected"> Loading QuickBooks Connect button </div> <div class="controls" ng-show="connected"> <connect-to-quickbooks /> </div> </div> </div>
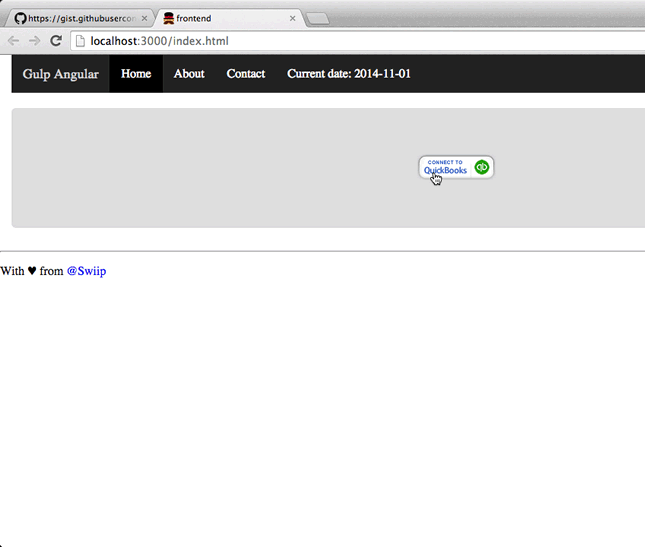
Handling external Javascript
There a few other ways to handle external Javascript dependencies in Angular but I like using the onload attribute in combination with Angular events as it is simple and easy to follow. In this refactoring I was able to save quite a few lines of code while improving read-ability.
- Pushed on 11/03/2014 by Christian
- QuickBooks Integration Consulting